Questions searched on google
Serilog writing to Database
Creating Serilog Table from EF Core
XML Type EF Core
Data Annotation XML type
Adding Serilog in .NET Core 3
We will use the approach of Entity Framework Core Generated table for Serilog Logging in Database.
First create a Log Entity class in your Models folder with code below code
public class Log
{
public int Id { get; set; }
public string Message { get; set; }
public string MessageTemplate { get; set; }
public string Level { get; set; }
public DateTime TimeStamp { get; set; }
public string Exception { get; set; }
[Column(TypeName ="Xml")]
public string Properties { get; set; }
public string LogEvent { get; set; }
}
Now goto your context class file and the following code
public virtual DbSet<Log> Logs { get; set; }
and add the below line in OnModelCreating method
modelBuilder.Entity<Log>().ToTable("Log");
Now we have defined the details of table which we want entity framework to genereate in database. Open the powershell and run the migrations command as below
dotnet ef migrations add Log --context AppContext
Check the migrations file name <timestamp>_Log.cs with the required changes we need and then update the database
dotnet ef database update --context AppContext
Now check the SQL Server Object Explorer with the updated database . A table exists named Log
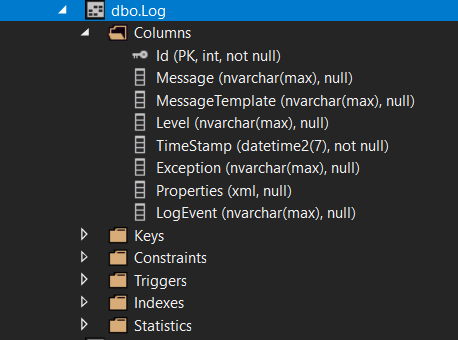
So we now have the required table for Serilog to begin logging . Now we will configure the serilog for this . Open nuget package manager console and add the below package in order to enable the Serilog sink for MSSqlServer
Serilog.Sinks.MSSqlServer
Add below package in order to activate the serilog configuration reader feature which will read how to connect to MSSqlServer
Serilog.Settings.Configuration
Install-Package Serilog.Sinks.MSSqlServer
Install-Package Serilog.Settings.Configuration
Now we have the basic packages installed , we will add the appsettings for the serilog to connect to the MSSqlServer
Add the below code to your appsettings.json
{
"Serilog": {
"Using": [ "Serilog.Sinks.MSSqlServer" ],
"MinimumLevel": "Information",
"WriteTo": [
{
"Name": "MSSqlServer",
"Args": {
"connectionString": "Server=(localdb)\\mssqllocaldb;Database=DatabaseName;Trusted_Connection=True;MultipleActiveResultSets=true", // connection String
"tableName": "Log" // table name
}
}
]
}
}
We have kept the table name as "Log" same as we have defined in the AppContext class for entity framework core to create in database server.
Now add the following code in your Program.cs
public class Program
{
public static void Main(string[] args)
{
var configuration = new ConfigurationBuilder()
.AddJsonFile("appsettings.json")
.Build();
Log.Logger = new LoggerConfiguration()
.ReadFrom.Configuration(configuration)//Serilog.Settings.Configuration
.WriteTo.Console()
.CreateLogger();
try
{
Log.Information("Getting the Application Clanstech Application Running... ");
CreateHostBuilder(args).Build().Run();
}
catch(Exception ex)
{
Log.Fatal(ex, "Host terminated unexpectedly");
}
finally
{
Log.CloseAndFlush();
}
}
public static IHostBuilder CreateHostBuilder(string[] args) =>
Host.CreateDefaultBuilder(args)
.UseSerilog()//using Serilog.AspNetCore
.ConfigureWebHostDefaults(webBuilder =>
{
webBuilder.UseStartup<Startup>();
}).UseDefaultServiceProvider(options => options.ValidateScopes = false);
}
Before using .UseSerilog() method do remember to install the package
Install-Package Serilog.AspNetCore
Now run your application and you will see all the logs populated in your Log Table in database server .
References :
https://www.carlrippon.com/asp-net-core-logging-with-serilog-and-sql-server/
https://www.c-sharpcorner.com/article/file-logging-and-ms-sql-logging-using-serilog-with-asp-net-core-2-0/
https://nblumhardt.com/2019/10/serilog-in-aspnetcore-3/
2894b87c-96f8-46bc-84da-ba159a3521a2|0|.0|96d5b379-7e1d-4dac-a6ba-1e50db561b04
ASP.NET MVC Core, C#, Logging, MSSQL, Serilog
Logging, Logging TO Database, Serilog, ASP.NET MVC Core, C#, MSSQL Server